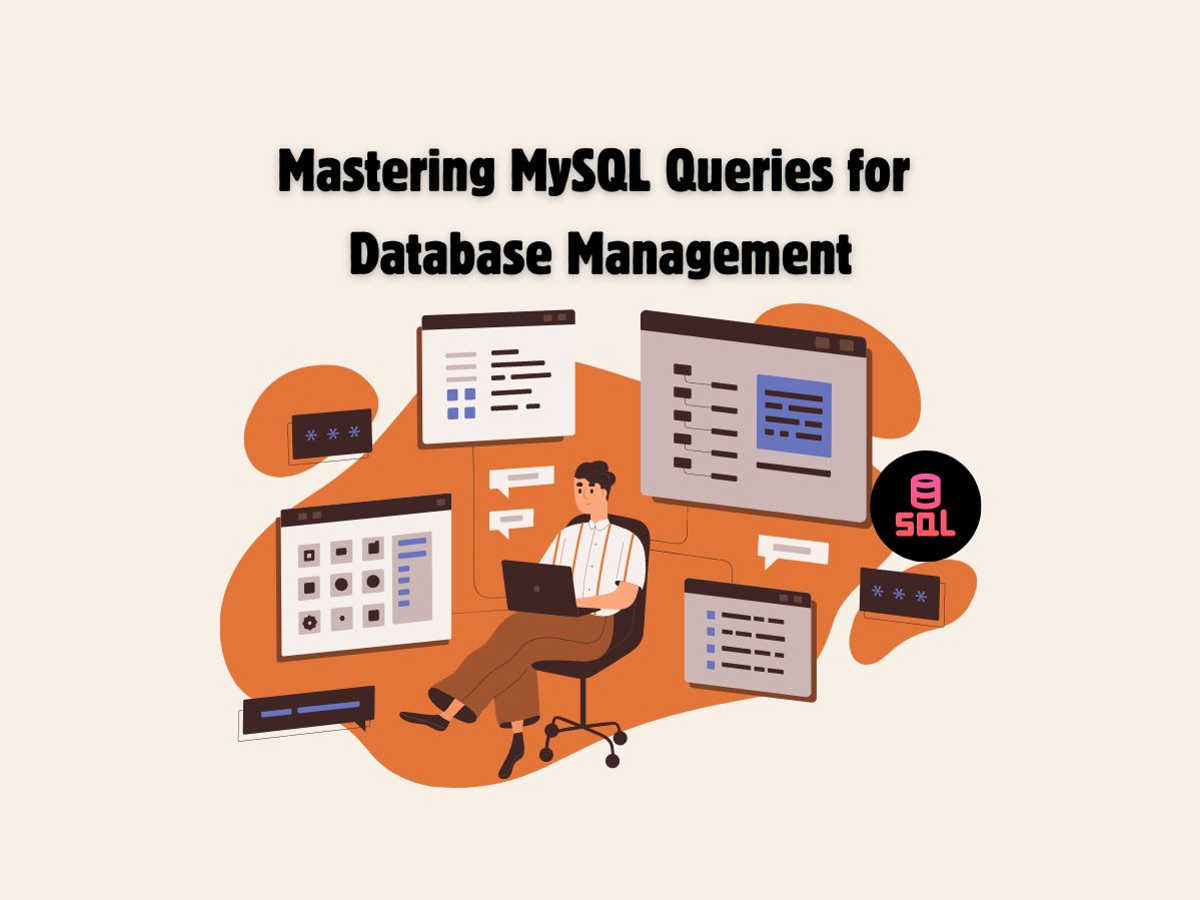
Learning MySQL Queries for Effective Database Management
MySQL is a powerful, open-source relational database management system (RDBMS) widely known for its reliability, performance, and ease of use. When managing a MySQL database, it is crucial to learn essential queries to create and maintain efficient data systems. In this blog post, we will discuss various MySQL queries and their usage to help you gain better control of your database operations.
1. Connecting to a MySQL Database
Before you can execute any queries, you need to connect to the MySQL database using a client such as MySQL Workbench, phpMyAdmin, or the MySQL command-line interface (CLI). You will need the following information:
Database hostname (usually `localhost` or a remote IP address)
Username
Password
Data store name
For example, when using the MySQL CLI, use the following command to connect:
mysql -h [hostname] -u [username] -p[password]
2. Selecting a Database and Tables
After connecting to the MySQL server, select a specific database before executing queries:
USE database_name;
To view the list of tables in the selected database, use:
SHOW TABLES;
3. Creating and Managing Tables
A well-structured table is the backbone of database management. To create a table, use the `CREATE TABLE` statement along with the required columns and their data types:
CREATE TABLE table_name ( column1 datatype, column2 datatype, …);
For example:
CREATE TABLE users ( id INT AUTO_INCREMENT PRIMARY KEY, username VARCHAR(255) NOT NULL, email VARCHAR(255) NOT NULL, created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP);
To drop or delete a table, use `DROP TABLE`. **Be cautious with this command, as it will delete the table and all associated data**:
DROP TABLE table_name;
4. Inserting Data into Tables
To add data to your table, use the `INSERT INTO` statement and specify the columns and values:
INSERT INTO table_name (column1, column2, …) VALUES (value1, value2, …);
For example:
INSERT INTO users (username, email) VALUES (‘JohnDoe’, ‘[email protected]’);
5. Retrieving Data from Tables
The `SELECT` statement is used to query data from tables. For example, to select all columns and rows:
SELECT * FROM table_name;
To retrieve specific columns, specify the column names:
SELECT column1, column2 FROM table_name;
Use the `WHERE` clause to filter data based on a condition:
SELECT * FROM table_name WHERE column1 = value;
To sort results, use the `ORDER BY` clause:
SELECT * FROM table_name ORDER BY column1 ASC;
6. Updating Data in Tables
To modify existing data in a table, use the `UPDATE` statement along with a `WHERE` clause to find the row(s) that need modifying:
UPDATE table_name SET column1 = value1, column2 = value2 WHERE condition;
For example:
UPDATE users SET email = ‘[email protected]’ WHERE username = ‘JohnDoe’;
7. Deleting Data from Tables
The `DELETE` statement allows you to remove specific data from a table. Remember to use a `WHERE` clause to identify the specific row(s) that should be deleted:
DELETE FROM table_name WHERE condition;
For example:
DELETE FROM users WHERE username = ‘JohnDoe’;
This brief introduction to MySQL queries is just scratching the surface of effective database management. As you become more familiar with MySQL commands, you will gain more control and efficiency in managing your databases. To further enhance your skills, consider diving into more advanced topics such as `JOIN`, `GROUP BY`, indexing, and stored procedures.